Angular From Scratch Tutorial – Index
PREVIOUS: Angular From Scratch Tutorial – Step 6: Service And Dependency Injection
Table of Contents
TARGET
Revision for working with forms when you have already worked with Angular but after some time you need to refresh your memory.
The theory comes from Introduction to forms in Angular doc.
This page is a summary for fast revision.
If it is your first time, you’d better go to Angular Documentation.
Summary
One of the advantages of working directly with form control objects is that value and validity updates are always synchronous and under your control.
Angular offers two form-building technologies: reactive forms and template-driven forms.
These two belong to the @angular/forms library and share a series of form control classes.
They even have their own modules: ReactiveFormsModule and FormsModule.
Reactive forms provide synchronous access to the data model, immutability with observable operators, and change tracking through observable streams.
Template-driven forms let direct access modify data in your template, but are less explicit than reactive forms because they rely on directives embedded in the template, along with mutable data to track changes asynchronously.
@SEE: more at:
Learn Angular with free step by step angular tutorials [ML44649]
Choosing an approach
Reactive forms and template-driven forms process and manage form data differently. Each approach offers different advantages.
FORMS | DETAILS |
---|---|
Reactive forms | Provide direct, explicit access to the underlying forms object model. Compared to template-driven forms, they are more robust: they’re more scalable, reusable, and testable. If forms are a key part of your application, or you’re already using reactive patterns for building your application, use reactive forms. |
Template-driven forms | Rely on directives in the template to create and manipulate the underlying object model. They are useful for adding a simple form to an app, such as an email list signup form. They’re straightforward to add to an app, but they don’t scale as well as reactive forms. If you have very basic form requirements and logic that can be managed solely in the template, template-driven forms could be a good fit. |
Key differences
Common form foundation classes
Template-driven models
import { Component, OnInit } from '@angular/core'; import { FormControl } from '@angular/forms'; @Component({ selector: 'app-forms2', template: ` Favorite Color: <input type="text" [(ngModel)]="favoriteColor"> `, styleUrls: ['./forms2.component.sass'] }) export class Forms2Component implements OnInit { favoriteColor = ''; constructor() { } ngOnInit(): void { } }
Reactive forms
import { Component, OnInit } from '@angular/core'; import { FormControl } from '@angular/forms'; @Component({ selector: 'app-forms1', template: ` Favorite Color: <input type="text" [formControl]="favoriteColorControl"> `, styleUrls: ['./forms1.component.sass'] }) export class Forms1Component implements OnInit { favoriteColorControl = new FormControl(''); constructor() { } ngOnInit(): void { } }
Additional Links
Setup
>PROBLEM
Running angular using ngModels, you get:
error NG8002: Can’t bind to ‘formControl’ since it isn’t a known property of ‘input’.
Example, implementing the following code:
You get:
>SOLUTION
There are at least two things to be observed.
1. Require modules setup.
Set the required modules in app.modules.ts:
import { FormsModule, ReactiveFormsModule } from “@angular/forms”;
…
@NgModule({
declarations: [
//…
],
imports: [
//…
FormsModule,
ReactiveFormsModule,
],
*** IMPORTANT NOTE:
If you are using a page under its specific module, declare in that module two.
Example:
app
+–forms-lab
+– forms1
— forms-lab.module.ts
Edit forms-lab.module.ts and declare both modules referred to above.
2. Make sure that you have not overwritten the angular modules:
It is something easy to happen, for instance, if you create a module called forms, as it is:
ng g m forms
This command will generate a FormsModule that overrides the angular’s module.
This conflict causes the same issue.
If it happened, delete, and recreate it with another name.
@SEE:
>ENV
Angular CLI: 13.2.2
Node: 16.13.1
Package Manager: npm 8.5.4
OS: win32 x64
Accessing field values with ngForm
<h6><a href="https://angular.io/api/forms/NgForm">https://angular.io/api/forms/NgForm</a></h6> <form #itemForm="ngForm" (ngSubmit)="onSubmit(itemForm)"> <label for="name">Name</label> <input type="text" id="name" class="form-control" name="name" ngModel required /> <button type="submit">Submit</button> </form> import {NgForm, FormGroup} from '@angular/forms'; onSubmit(f: NgForm): void { console.log(f.value); // { first: '', last: '' } console.log(f.valid); // false alert(f.value.emailid); }
Referencing Snippets
>snippet #1 - .ts file: // convenience getter for easy access to form fields get f() { return this.loginForm.controls; } - .html file: <div *ngIf="submitted && f.username.errors" class="invalid-feedback"> <div *ngIf="f.username.errors.required">Username is required</div> </div> >snippet #2 - .html file: <form *ngIf="!isLoggedIn" name="form" (ngSubmit)="f.form.valid && onSubmit()" #f="ngForm" novalidate >
import {Component} from '@angular/core'; import {NgForm} from '@angular/forms'; @Component({ selector: 'example-app', template: ` <form #f="ngForm" (ngSubmit)="onSubmit(f)" novalidate> <input name="first" ngModel required #first="ngModel"> <input name="last" ngModel> <button>Submit</button> </form> <p>First name value: {{ first.value }}</p> <p>First name valid: {{ first.valid }}</p> <p>Form value: {{ f.value | json }}</p> <p>Form valid: {{ f.valid }}</p> `, }) export class SimpleFormComp { onSubmit(f: NgForm) { console.log(f.value); // { first: '', last: '' } console.log(f.valid); // false } }
ngSubmit
SEE:
– Build angular 13 forms with example [ML44183]
– Angular: FormGroupDirective
Snippets
- .html file: <form *ngIf="!isLoggedIn" name="form" (ngSubmit)="f.form.valid && onSubmit()" #f="ngForm" novalidate > - .ts file: onSubmit(): void { const { username, password } = this.form; this.authService.login(username, password).subscribe({ next: data => { this.tokenStorage.saveToken(data.accessToken); this.tokenStorage.saveUser(data); this.isLoginFailed = false; this.isLoggedIn = true; this.roles = this.tokenStorage.getUser().roles; this.reloadPage(); }, error: err => { this.errorMessage = err.error.message; this.isLoginFailed = true; } }); }
<select>
>component.ts activities: any; activity: string; constructor( private activityService: ActivityService, private todosService: TodosService, ) { activityService.load(this.email, this.stoken).subscribe((objs) => { this.activities = []; for (let i = 0; i < objs.activities.length; i++) { this.activities[i] = objs.activities[i]; } }); this.activity = 'all activities'; } newTodoSubmit(): void { alert(this.activity); } >component.html <select id="activities_id" class="form-select caret" [(ngModel)]="activity" style="border-color: transparent !important; border-width: 0px !important; margin-top: 0px; font-size: 12px !important; width: 120px !important;"> <option value="{{activity}}" selected>all activities</option> <option value="{{activity.name}}" *ngFor="let activity of activities">{{activity.name}}</option> </select>
Angular From Scratch Tutorial – Index
PREVIOUS: Angular From Scratch Tutorial – Step 6: Service And Dependency Injection
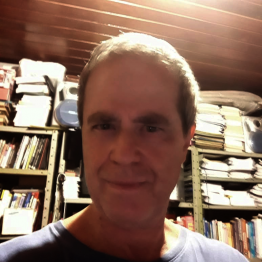
Brazilian system analyst graduated by UNESA (University Estácio de Sá – Rio de Janeiro). Geek by heart.