IMPORTANT NOTE
The procedures described here details how the application was created.
Follow them if you have created your application from scratch, otherwise it is not necessary since it is already done.
In both cases, the procedures are useful to understand the details.
Table of Contents
#DOWNLOAD
Download the project at Github from “features/step02” branch.
#CREATING MODULE
– Create the module for the 1st step denoted by stepone:
ng g module steptwo
#CREATING THE ANGULAR COMPONENTS
ng g c home
ng g c steptwo/steptwo
– register into app.module.ts:
import { SteptwoModule } from ‘./steptwo/steptwo.module’;
imports: [
…
SteptwoModule,
…
]
#ROUTING
Edit app-routing.module.ts.
Add as follows:
import { SteptwoModule } from './steptwo/steptwo.module';
const routes: Routes = [
{path: '', component: HomeComponent, pathMatch: 'full'},
{path: 'steptwo', component: SteptwoComponent},
{path: '**', component: HomeComponent}
];
@NgModule({
imports: [
RouterModule.forRoot(routes),
SteponeModule,
],
exports: [RouterModule]
})
#VIEW ADJUSTMENTS
Copy the index.html file to your project.
Edit app-component.html and replace all the content with just this:
#HOW DOES IT WORK?
When pointed to localhost:4200 (or another port), the routing service will redirect to the default address using the router’s empty declaration that points to HomeComponent.
This is configured in the app-routing.module.ts file at:
const routes: Routes = [ {path: '', component: HomeComponent, pathMatch: 'full'}, // router's empty declaration // ... {path: '**', component: HomeComponent} // router's any other declaration ];
That way, the index.html file contains the common features shared with all the application’s pages.
Similarly, the app.component.html file also shares, but unlike index.html, the app.component.html may add extra processing resources using its app.component.ts file.
#IMPLEMENTING NAVBAR BOOTSTRAP COMPONENT
The home.component.html requires a navbar.
Unfortunately, it is not possible to use Bootstrap’s dynamic features, like opening and closing events, straight from its library.
The first issue that you notice is that navbar fails.
Dynamic features requires Ng-bootstrap behind the scene that it is used to implement a dynamic bootstrap component in the Angular world.
Run the commands to create a component module and its component:
ng g module components
ng g c components/navbar
Replace the component module created above replacing it with the respective code from Github from “features/step02” branch.
Why is the component replaced and not just copied?
We need to run the commands to create it because of the additional registering actions performed during this operation, then we may replace contents.
#REGISTERING THE COMPONENT
Edit app.module.ts and register the module and the component.
A module is imported and a component decrared.
@NgModule({
declarations: [
AppComponent,
HomeComponent
],
imports: [
BrowserModule,
AppRoutingModule,
NgbModule,
SteptwoModule,
ComponentsModule
],
providers: [],
bootstrap: [AppComponent]
})
If the component module is not registered, the selector element is not recognized generating error message.
Now edit app.component.html and add the navbar selector:
Remember that everything added to app.component.html will be shared with all pages.
#Navbar closed
#Navbar Opened
#IMPLEMENTING STEP TWO PAGE
#PAGE DEFAULT CONTENT
When a component is created, by default you get a “component works” text like shown below.
#IMPLEMENTING STEPTWO PAGE CONTENT USING BOOTSTRAP
Now, the easiest part.
To add Bootstrap code is a very simple operation.
In this page, it was used the Jumbotron example from Boostrap site.
The trick is that you copy just part of the code, since the basic structure is already defined by index.html, app.component.html, etc.
Open the steptwo.component.html at Github from “features/step02” branch and copy its content to past to your project’s page.
Notice that it was used just the new code to be used in the page, discarding the scaffolding code.
This is really good because templates reduce maintenance.
This is the page you get from the tutorial.
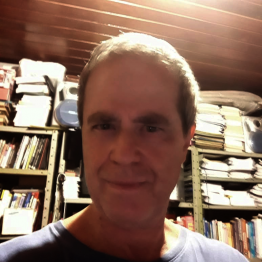
Brazilian system analyst graduated by UNESA (University Estácio de Sá – Rio de Janeiro). Geek by heart.